Radar Plot¶
Let us start generating some data:
[1]:
import numpy as np
np.random.seed(3)
ideal_point = np.array([0.15, 0.1, 0.2, 0.1, 0.1])
nadir_point = np.array([0.85, 0.9, 0.95, 0.9, 0.85])
F = np.random.random((1, 5)) * (nadir_point - ideal_point) + ideal_point
print(F)
[[0.53555853 0.66651826 0.41817855 0.50866208 0.76971022]]
If the values should not be normalized, then we can plot the ideal and nadir point. This keeps the absolute values of each objective. The outer shape represents the nadir point, the inner area the ideal point. All points will lie in the area spanned by those two points additionally.
[2]:
from pymoo.visualization.radar import Radar
plot = Radar(bounds=[ideal_point, nadir_point], normalize_each_objective=False)
plot.add(F)
plot.show()
[2]:
<pymoo.visualization.radar.Radar at 0x10444c6b0>
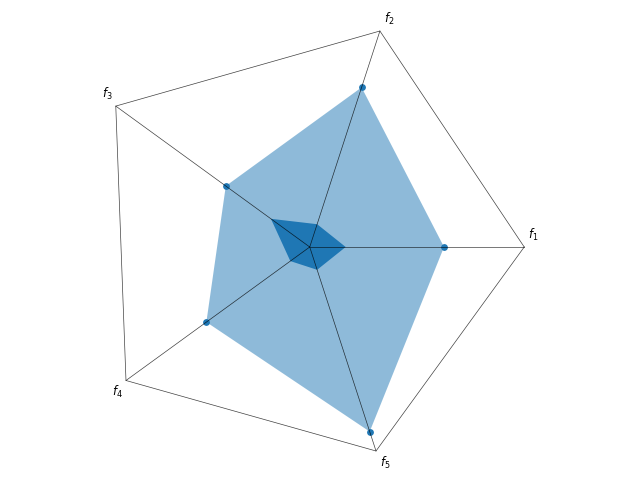
But if the scale of the objective is too different, then normalization is recommended. Then, the ideal point is just the point in the middle, and the nadir point is now symmetric.
[3]:
plot = Radar(bounds=[ideal_point, nadir_point])
plot.add(F)
plot.show()
[3]:
<pymoo.visualization.radar.Radar at 0x1048b8a40>
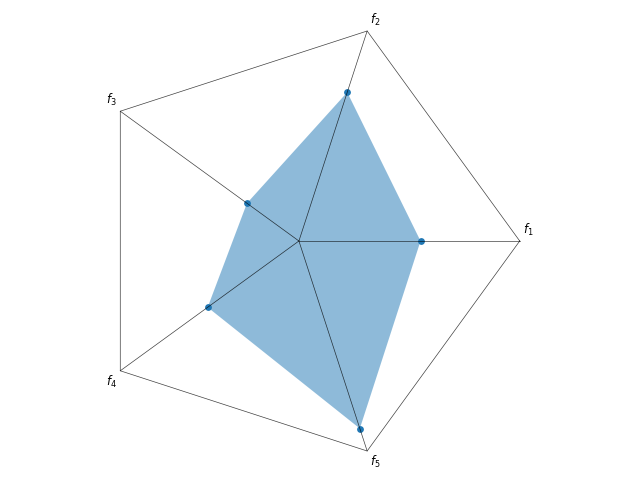
[4]:
F = np.random.random((6, 5)) * (nadir_point - ideal_point) + ideal_point
plot = Radar(bounds=[ideal_point, nadir_point],
axis_style={"color": 'blue'},
point_style={"color": 'red', 's': 30})
plot.add(F[:3], color="red", alpha=0.8)
plot.add(F[3:], color="green", alpha=0.8)
plot.show()
[4]:
<pymoo.visualization.radar.Radar at 0x117aa1160>
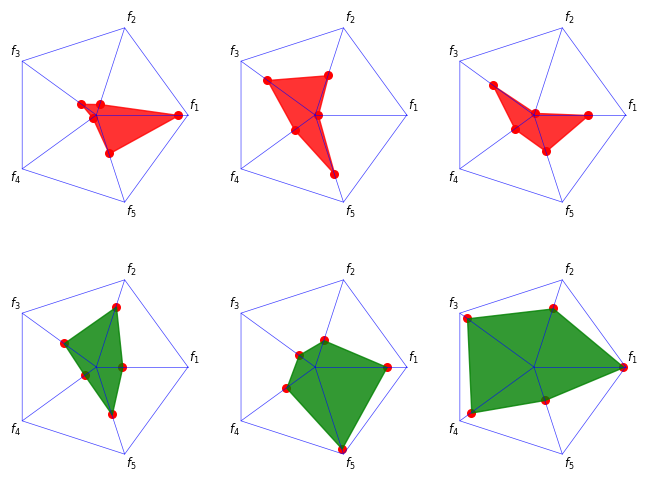
API¶
-
class
pymoo.visualization.radar.
Radar
(self, normalize_each_objective=True, n_partitions=3, point_style={}, **kwargs) Radar Plot
- Parameters
- normalize_each_objectivebool
Whether each objective is normalized. Otherwise, the inner and outer bound is plotted.
- point_styledict
The style being used to visualize the points
- n_partitionsint
Number of partitions to show in the radar.
- reversebool
If plot requires normalization, then the reverse values can be plotted (1 - Input). For some plots it can be useful to interpret a larger area as better regarding a value. If minimization applies, a smaller area means better, which can be misleading.
- axis_styledict
Most of the plots consists of an axis. The style of the axis, e.g. color, alpha, …, can be changed to further modify the plot appealing.
- labelsstr or list
The labels to be used for each variable provided in the plot. If a string is used, then they will be enumerated. Otherwise, a list equal to the number of variables can be provided directly.
- Other Parameters
- figsizetuple
The figure size. Default (figsize=(8, 6)). For some plots changing the size might have side effects for position.
- titlestr or tuple
The title of the figure. If some additional kwargs should be provided this can be achieved by providing a tuple (“name”, {“key” : val}).
- legendstr
Whether a legend should be shown or not.
- tight_layoutbool
Whether tight layout should be used.
- cmapcolormap
For some plots different kind of colors are used. The colormap can be changed to modify the color sequence for the plots.